Motivations
I wanted to explore consensus algorithms like Paxos and Raft, and delve into Golang channels. Additionally, I aimed to create a project that would leverage concurrency and behavior driven design (BDD)
, allowing me to deepen my understanding of these concepts.
Tech Stack
-
Go
Raft consensus
Concurrency
Behavior Driven Design
Architecture
High Level
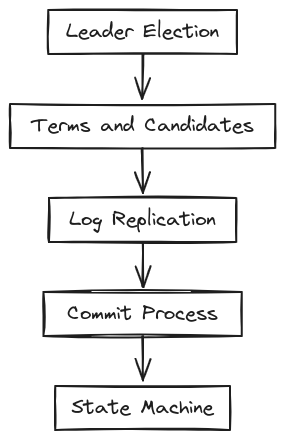
This diagram outlines the main stages of the Raft consensus algorithm:
Leader Election
: Nodes in the Raft cluster participate in leader election based on terms and candidates.Terms and Candidates
: Nodes exchange information to determine the leader for a specific term.Log Replication
: The leader replicates its log entries to other nodes in the cluster.Commit Process
: Once a majority of nodes acknowledge the log entry, it is committed.State Machine
: Committed log entries are applied to the state machine, ensuring consistent state across the cluster.
Component Level flows
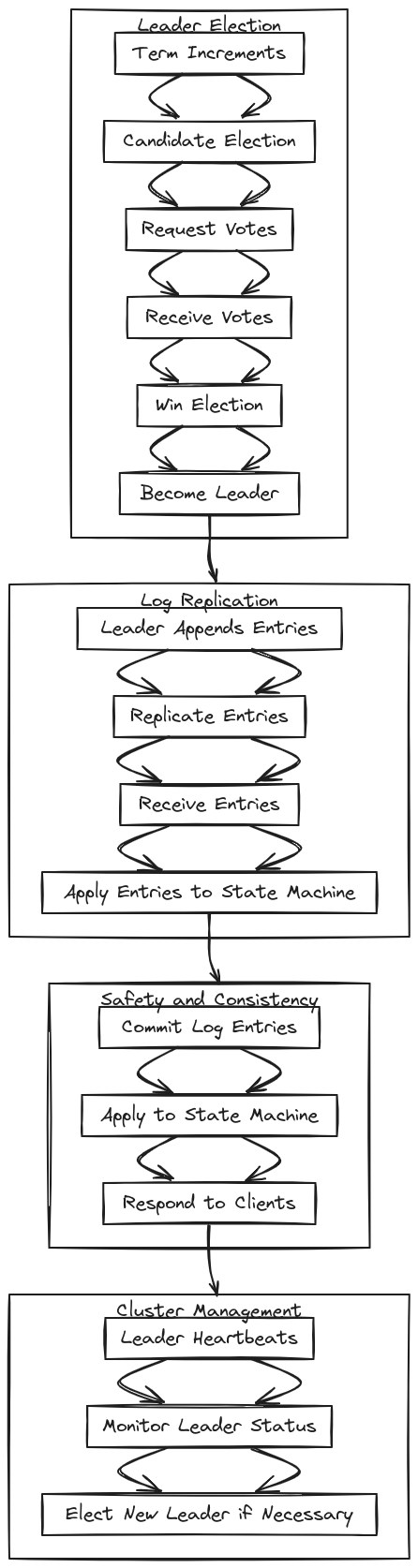
This detailed diagram illustrates the comprehensive process of the Raft consensus algorithm:
Leader Election
: Nodes increment terms, initiate candidate elections, request and receive votes, win elections, and become leaders.Log Replication
: The leader appends log entries, replicates them to followers, and followers receive and apply these entries to their state machines.Safety and Consistency
: Committed log entries are confirmed, applied to state machines, and responses are sent to clients, ensuring data safety and consistency.Cluster Management
: Leaders send heartbeats, monitor leader status, and initiate new leader elections if necessary to maintain cluster stability and leadership integrity.
Behavior Driven Design & SOLID principles
Behavior Driven Design
(BDD) focuses on defining software behavior through examples and aligning development with user needs. When implementing the Raft consensus algorithm in Golang independently, I applied BDD principles by using Golang’s test primitives extensively. These primitives allowed me to articulate and verify every aspect of the algorithm’s behavior, including leader election, log replication, and safety mechanisms. Each component was systematically tested with examples that covered expected outcomes and edge cases, ensuring that the implementation met technical specifications and functional requirements effectively. By adopting BDD principles in this solo project, I ensured clarity in requirements, robustness in implementation, and alignment with the intended functionality of the Raft consensus algorithm in Golang.
In applying BDD to design the Raft consensus algorithm, I ensured adherence to SOLID
principles, particularly focusing on the Dependency Inversion Principle
. This principle guided me to design components where high-level modules depended on abstractions rather than concrete implementations. For instance, I abstracted interfaces for key Raft components. By doing so, I enabled flexibility and easier testing, allowing different implementations to be substituted without affecting the overall system behavior. This approach not only enhanced modularity but also facilitated easier implementation of tests.